Posted in ruby, scripting macs | Monday, December 17th, 2007 | Trackback
I wanted to write a script that used Growl to notify a user. That user could click on the Growl sidle-up, and that would make the script do something. It took me a while to figure out callbacks from Growl to the originating app, so I thought I’d write it down.
There are a couple of Growl libraries out there, but the code that best suited my needs comes from Satoshi Nakagawa’s LimeChat. Since it’s not packaged separately, I include it here.
A bit more detail on the proof-of-concept app. The first balloon looks like this:
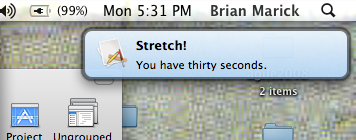
When I click on it, the app pops up another notification and exits:
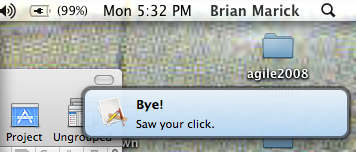
Here’s the code:
require ‘osx/cocoa‘
include OSX
require ‘growl‘
class GrowlController
PING_KIND = ‘stretch‘
ACK_KIND = ‘ack‘
def initialize
@growl = Growl::Notifier.alloc.initWithDelegate(self)
@growl.start(:this_program, [PING_KIND, ACK_KIND])
end
def ping
@growl.notify(kind = PING_KIND,
title = ‘Stretch!‘,
description = ‘You have thirty seconds.‘,
context = "new", sticky = true, priority = 0)
end
def growl_onClicked(sender, context)
@growl.notify(kind = ACK_KIND,
title = ‘Bye!‘,
description = ‘Saw your click.‘,
context = nil, sticky = true, priority = 0)
exit!
end
end
if $0 == __FILE__ then
growl_controller = GrowlController.new
growl_controller.ping
NSApplication.sharedApplication
NSApp.run
end
|
December 19th, 2007 at 7:05 pm
[…] Safari. It’ll also show you how to write code that makes use of Mac framework features (like sending Growl notifications and putting controllers for your scripts in the status bar). It won’t be your definitive […]